Drupal Coding Standards
Adhering to specific programming standards while creating websites is key to project quality, security, and scalability. In this article, we will discuss the essence of these standards and show practices that allow for effective function implementation on the Drupal platform.
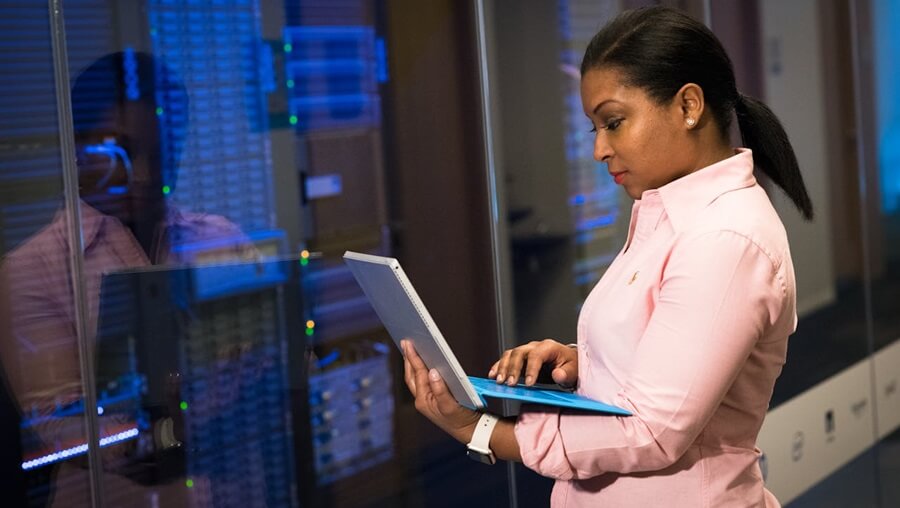
What Are the Coding Standards in Drupal?
What Are the Coding Standards in Drupal?
Drupal coding standards refer to rules, conventions, and practices defining how you should write code. They provide guidelines for:
- structure,
- formatting,
- documenting the code.
The standards are designed to facilitate developers' collaboration and ensure the source code's readability and consistency. In short, coding standards in Drupal measure quality and professionalism in creating web applications based on this system.
Basic Programming Standards Implementation Rules
Basic Programming Standards Implementation Rules
Essential rules comprise using uniform code formatting, including appropriate indentation, brackets, and spacing. It is also crucial to properly document the code through comments that describe its operation and the meaning of particular fragments.
Moreover, a key principle is to avoid direct changes to the core system files, which ensures easier updates and platform maintenance. A good example is using hooks to modify the system’s behavior without interfering with its core files.
Coding Standards in Different Programming Languages
Coding Standards in Different Programming Languages
Drupal code standards are fixed. This means they are independent of the Drupal version you are working on. New code should adhere to the latest rules to ensure consistency and readability. Learn more about Drupal coding standards on the official system’s website.
PHP Coding Standards in Drupal
PHP code standards are based on PEAR. Here are the most crucial ones:
1. Use 2-space indentation, no tabs.
2. Lines should not contain trailing whitespace.
3. All blocks at the beginning of the PHP file should be separated by a blank line.
The heading can look as follows:
<?php namespace This\Is\The\Namespace; use Drupal\foo\Bar; /** * Provides examples. */ class ExampleClassName {
4. A single code line should not contain over 80 characters, except for lines containing longer function names, function/class definitions, variable declarations, etc.
5. Function names should be written in lowercase and an underscore should separate words. Functions should contain group/module names as a prefix to avoid name clashes between modules.
6. Variables should be named in lowercase, and words should be separated by uppercase letters (example: $lowerCamelCase) or underscores (example: $snake_case). Be consistent and do not mix camelCase and snake_case variable names.
Operators
All binary operators, such as +, -, =, !=, ==, >, etc., should have a space before and after the operator. For example, an assignment should be formatted as $foo = $bar.
Unary operators (e.g., ++) should not contain spaces between the operator and the variable or number they operate on.
Conditional Statements and Loops
Control statements should contain one space between the keyword and the opening bracket to distinguish them from function triggers.
Always use curly brackets in control statements, even when they are optional. They improve readability and reduce the likelihood of errors when adding new lines.
- The opening curly bracket should be in the same verse as the opening statement, preceded by one space.
- The closing curly bracket should be added on a separate line and indented to the same level as the opening statement.
Exemplary if else statement structure:
if (condition1 || condition2) { action1; } elseif (condition3 && condition4) { action2; } else { defaultaction; }
Exemplary switch statement structure:
switch (condition) { case 1: action1; break; case 2: action2; break; default: defaultaction; }
Exemplary while loop statement:
do { actions; } while ($condition);
Function Triggering
Functions must be activated without spaces separating the function name from the opening bracket and first parameter, with no spaces between commas and each parameter, and with no spaces between the last parameter, the closing bracket, and the semicolon. Here is an example:
$var = foo($bar, $baz, $quux);
Arrays
Arrays are declared using a short syntax with spaces separating each element after a comma. If there is an assignment operator =>, you must add a space before and after the operator, e.g.:
$array = ['hello', 'world', 'foo' => 'bar'];
If the array declaration exceeds 80 characters, you must divide it into several lines, e.g.:
$form['title'] = [ '#type' => 'textfield', '#title' => t('Title'), '#size' => 60, '#maxlength' => 128, '#description' => t('The title of your node.'), ];
JavaScript Coding Standards in Drupal
Learn the crucial rules when it comes to JavaScript programming standards in Drupal.
Drupal Behaviors
In most cases, the JS code should be included in Drupal Behaviors – this is a JavaScript object that Drupal initializes after the page loads and after each AJAX trigger in the DOM (Document-Object-Model). Thanks to them, JavaScript integration in Drupal websites and projects becomes more convenient.
Indentation
As with PHP, you should use 2-space indentation, no tabs. Lines should not contain trailing whitespaces.
Semicolons
JavaScript allows for an optional “semicolon insertion” but it does not comply with Drupal standards. All statements (except function, if, switch, try, and while) MUST be terminated by a semicolon (;), and return values must start on the same line as the return keyword.
Naming
Variables and multi-word functions that are not constants or constructors should be named using the lowerCamelCase convention.
When a variable contains a jQuery object, it must start with a dollar sign ($):
var $form = $('#search-block-form'); var $inputs = $form.find('input');
Variables
All variables must be declared using var only once at the beginning of a function.
Each one should be declared in a separate line – including variables that are declared but do not receive a value, e.g.
var anArray = []; var eventCallback = function () {}; var curTableDragSetting;
Functions
The function name should start with a module or theme name declaring the function to avoid name clashes:
Drupal.behaviors.myModuleTableDrag = function (context) { Object.keys(Drupal.settings.tableDrag).forEach(function (base) { $('#' + base).once('tabledrag', addBehavior); }); };
Arrays
Arrays should be formatted with one space separating each element and operator:
var someArray = ['hello', 'world'];
Similarly to the PHP, if the array declaration exceeds 80 characters, you should divide into a few lines.
CSS Coding Standards in Drupal
Drupal CSS coding standards include recommendations for organizing styles, using CSS classes, and naming methodologies such as BEM (Block Element Modifier).
BEM Structure
Block Element Modifier helps keep the CSS code readable, consistent, and scalable, especially in large projects. It is based on three basic concepts: Block, Element, and Modifier.
1. A block is an independent element that can be used separately or as a part of a bigger structure. It is a main container that contains many elements with a single function. Blocks have unique names and represent large parts of a website such as headers, navigation menus, footers, e.g.:
.navbar { /* Styles for navbar block */ }
2. An element is part of a block that depends on it. It should not be used on its own outside the context of that block. Elements are usually marked with classes within the block and used to define individual parts. e.g.:
.navbar__item { /* Styles for navbar block item */ }
3. A modifier is an additional class that changes the appearance and behavior of a block or element. This allows for flexibility in styling while maintaining consistency. Modifiers can change the size, color, hide, or add functions, such as:
.navbar--dark { /* Modifier changing the navbar style to dark */ } .navbar__item--active { /* Modifier highlighting the active element in the navbar */ }
Defining Components in Their CSS Files
It is best to place the components in their own CSS files and load them only when necessary. Avoid mixing multiple components in one file. This will keep the code clear and manageable.
Formatting CSS in Drupal
Consider these essential guidelines for formatting CSS in Drupal:
- Use 2 spaces for every indentation level;
- Declarations must be indented one level from the selector;
- Do not leave trailing whitespaces at the end of the line;
- End all text files with a single blank line;
- Place a colon directly before the property name, followed by a single space and the property value;
- Terminate all declarations with a semicolon.
Programming Standards – Twig
Twig is a template engine used in Drupal to generate dynamic HTML content. It is based on PHP syntax, but it is safer and easier. The most important aspects of twig programming standards are:
- Adding only one space after the start of the delimiter (“{{“,” {%”,” {#”) and before the end of it (“}}”,” %}”,” #”}).
- Adding one space before and after comparison, mathematical, logical, ~, is, in, and ternary operators.
- Adding one space in arrays and hashes.
- Not adding a space after an open bracket or before a closed bracket in expressions.
- Not adding spaces before and after string delimiters.
- Not adding spaces to operators like “|”,” .”,” ..”,” []”.
- Avoiding spaces before and after brackets used for filter and function triggering.
- Using lowercase variables and separating them with an underscore.
- Using the same indentation as the target language of the template being rendered.
Useful Tools for Formatting Code According to Standards
Useful Tools for Formatting Code According to Standards
Various code formatting tools have been introduced to ensure code consistency and quality. This section will present 5 useful code formatting tools for PHP, JavaScript, and CSS, often used in web development.
- PHPCS (PHP CodeSniffer) is a tool that automatically verifies your PHP code against specific standards. It analyzes your source code and highlights any violations of those standards.
- ESLint enables developers to create personalized linting rules and select from established standards like Airbnb, Google, or StandardJS. ESLint reviews JavaScript code for errors, style adherence, and performance issues.
- Stylelint is a CSS linting tool that helps keep your styles consistent and clean. It can be configured to apply a variety of rulesets, including popular standards like CSScomb, Sass Guidelines, and BEM. Stylelint analyzes CSS for errors, redundant properties, incorrect formatting, and browser independence.
- Twig CS Fixer – this tool helps automate fixing and keeping your Twig code clean by aligning it with established Twig programming standards.
- Coding standards Drupal – our team designed this tool. It contains extended configurations of other tools (e.g., PHP CodeSniffer, Coder, PHPStan, or Twig CS Fixer) to maintain high-quality code following established standards.
Summary
Summary
Adhering to Drupal coding standards is crucial for maintaining clean, readable, and efficient code. Following these guidelines promotes collaboration and enhances your website’s performance and security. If you have doubts or questions about this topic, contact a Drupal web development agency.